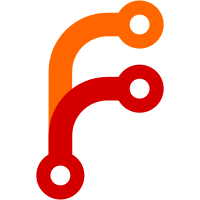
git-svn-id: svn://svn.code.sf.net/p/writer2latex/code/trunk@272 f0f2a975-2e09-46c8-9428-3b39399b9f3c
166 lines
7.5 KiB
Java
166 lines
7.5 KiB
Java
/************************************************************************
|
|
*
|
|
* W2XRegistration.java
|
|
*
|
|
* Copyright: 2002-2015 by Henrik Just
|
|
*
|
|
* This file is part of Writer2LaTeX.
|
|
*
|
|
* Writer2LaTeX is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation, either version 3 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* Writer2LaTeX is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with Writer2LaTeX. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
* Version 1.6 (2015-04-28)
|
|
*
|
|
*/
|
|
|
|
package org.openoffice.da.comp.writer2xhtml;
|
|
|
|
import com.sun.star.lang.XMultiServiceFactory;
|
|
import com.sun.star.lang.XSingleServiceFactory;
|
|
import com.sun.star.registry.XRegistryKey;
|
|
|
|
import com.sun.star.comp.loader.FactoryHelper;
|
|
|
|
/** This class provides a static method to instantiate our uno components
|
|
* on demand (__getServiceFactory()), and a static method to give
|
|
* information about the components (__writeRegistryServiceInfo()).
|
|
* Furthermore, it saves the XMultiServiceFactory provided to the
|
|
* __getServiceFactory method for future reference by the componentes.
|
|
*/
|
|
public class W2XRegistration {
|
|
|
|
public static XMultiServiceFactory xMultiServiceFactory;
|
|
|
|
/**
|
|
* Returns a factory for creating the service.
|
|
* This method is called by the <code>JavaLoader</code>
|
|
*
|
|
* @return returns a <code>XSingleServiceFactory</code> for creating the
|
|
* component
|
|
*
|
|
* @param implName the name of the implementation for which a
|
|
* service is desired
|
|
* @param multiFactory the service manager to be used if needed
|
|
* @param regKey the registryKey
|
|
*
|
|
* @see com.sun.star.comp.loader.JavaLoader
|
|
*/
|
|
public static XSingleServiceFactory __getServiceFactory(String implName,
|
|
XMultiServiceFactory multiFactory, XRegistryKey regKey) {
|
|
xMultiServiceFactory = multiFactory;
|
|
XSingleServiceFactory xSingleServiceFactory = null;
|
|
if (implName.equals(Writer2xhtml.__implementationName) ) {
|
|
xSingleServiceFactory = FactoryHelper.getServiceFactory(Writer2xhtml.class,
|
|
Writer2xhtml.__serviceName,
|
|
multiFactory,
|
|
regKey);
|
|
}
|
|
else if (implName.equals(W2XExportFilter.class.getName()) ) {
|
|
xSingleServiceFactory = FactoryHelper.getServiceFactory(W2XExportFilter.class,
|
|
W2XExportFilter.__serviceName,
|
|
multiFactory,
|
|
regKey);
|
|
}
|
|
else if (implName.equals(BatchConverter.__implementationName) ) {
|
|
xSingleServiceFactory = FactoryHelper.getServiceFactory(BatchConverter.class,
|
|
BatchConverter.__serviceName,
|
|
multiFactory,
|
|
regKey);
|
|
}
|
|
else if (implName.equals(XhtmlOptionsDialog.__implementationName)) {
|
|
xSingleServiceFactory = FactoryHelper.getServiceFactory(XhtmlOptionsDialog.class,
|
|
XhtmlOptionsDialog.__serviceName,
|
|
multiFactory,
|
|
regKey);
|
|
}
|
|
else if (implName.equals(XhtmlOptionsDialogMath.__implementationName)) {
|
|
xSingleServiceFactory = FactoryHelper.getServiceFactory(XhtmlOptionsDialogMath.class,
|
|
XhtmlOptionsDialogMath.__serviceName,
|
|
multiFactory,
|
|
regKey);
|
|
}
|
|
else if (implName.equals(XhtmlOptionsDialogCalc.__implementationName)) {
|
|
xSingleServiceFactory = FactoryHelper.getServiceFactory(XhtmlOptionsDialogCalc.class,
|
|
XhtmlOptionsDialogCalc.__serviceName,
|
|
multiFactory,
|
|
regKey);
|
|
}
|
|
else if (implName.equals(EpubOptionsDialog.__implementationName)) {
|
|
xSingleServiceFactory = FactoryHelper.getServiceFactory(EpubOptionsDialog.class,
|
|
EpubOptionsDialog.__serviceName,
|
|
multiFactory,
|
|
regKey);
|
|
}
|
|
else if (implName.equals(Epub3OptionsDialog.__implementationName)) {
|
|
xSingleServiceFactory = FactoryHelper.getServiceFactory(Epub3OptionsDialog.class,
|
|
Epub3OptionsDialog.__serviceName,
|
|
multiFactory,
|
|
regKey);
|
|
}
|
|
else if (implName.equals(EpubMetadataDialog.__implementationName)) {
|
|
xSingleServiceFactory = FactoryHelper.getServiceFactory(EpubMetadataDialog.class,
|
|
EpubMetadataDialog.__serviceName,
|
|
multiFactory,
|
|
regKey);
|
|
}
|
|
else if (implName.equals(ConfigurationDialog.__implementationName)) {
|
|
xSingleServiceFactory = FactoryHelper.getServiceFactory(ConfigurationDialog.class,
|
|
ConfigurationDialog.__serviceName,
|
|
multiFactory,
|
|
regKey);
|
|
}
|
|
else if (implName.equals(ToolbarSettingsDialog.__implementationName)) {
|
|
xSingleServiceFactory = FactoryHelper.getServiceFactory(ToolbarSettingsDialog.class,
|
|
ToolbarSettingsDialog.__serviceName,
|
|
multiFactory,
|
|
regKey);
|
|
}
|
|
|
|
return xSingleServiceFactory;
|
|
}
|
|
|
|
/**
|
|
* Writes the service information into the given registry key.
|
|
* This method is called by the <code>JavaLoader</code>
|
|
* <p>
|
|
* @return returns true if the operation succeeded
|
|
* @param regKey the registryKey
|
|
* @see com.sun.star.comp.loader.JavaLoader
|
|
*/
|
|
public static boolean __writeRegistryServiceInfo(XRegistryKey regKey) {
|
|
return
|
|
FactoryHelper.writeRegistryServiceInfo(BatchConverter.__implementationName,
|
|
BatchConverter.__serviceName, regKey) &
|
|
FactoryHelper.writeRegistryServiceInfo(Writer2xhtml.__implementationName,
|
|
Writer2xhtml.__serviceName, regKey) &
|
|
FactoryHelper.writeRegistryServiceInfo(W2XExportFilter.__implementationName,
|
|
W2XExportFilter.__serviceName, regKey) &
|
|
FactoryHelper.writeRegistryServiceInfo(XhtmlOptionsDialog.__implementationName,
|
|
XhtmlOptionsDialog.__serviceName, regKey) &
|
|
FactoryHelper.writeRegistryServiceInfo(XhtmlOptionsDialogMath.__implementationName,
|
|
XhtmlOptionsDialogMath.__serviceName, regKey) &
|
|
FactoryHelper.writeRegistryServiceInfo(XhtmlOptionsDialogCalc.__implementationName,
|
|
XhtmlOptionsDialogCalc.__serviceName, regKey) &
|
|
FactoryHelper.writeRegistryServiceInfo(EpubOptionsDialog.__implementationName,
|
|
EpubOptionsDialog.__serviceName, regKey) &
|
|
FactoryHelper.writeRegistryServiceInfo(Epub3OptionsDialog.__implementationName,
|
|
Epub3OptionsDialog.__serviceName, regKey) &
|
|
FactoryHelper.writeRegistryServiceInfo(EpubMetadataDialog.__implementationName,
|
|
EpubMetadataDialog.__serviceName, regKey) &
|
|
FactoryHelper.writeRegistryServiceInfo(ConfigurationDialog.__implementationName,
|
|
ConfigurationDialog.__serviceName, regKey) &
|
|
FactoryHelper.writeRegistryServiceInfo(ToolbarSettingsDialog.__implementationName,
|
|
ToolbarSettingsDialog.__serviceName, regKey);
|
|
}
|
|
}
|
|
|